layout: true --- class: middle .center[ # Networking TS w/Algorithms .accent[ ### ] ] ??? > * --- # Networking TS Workshop .center[ .image-60[ 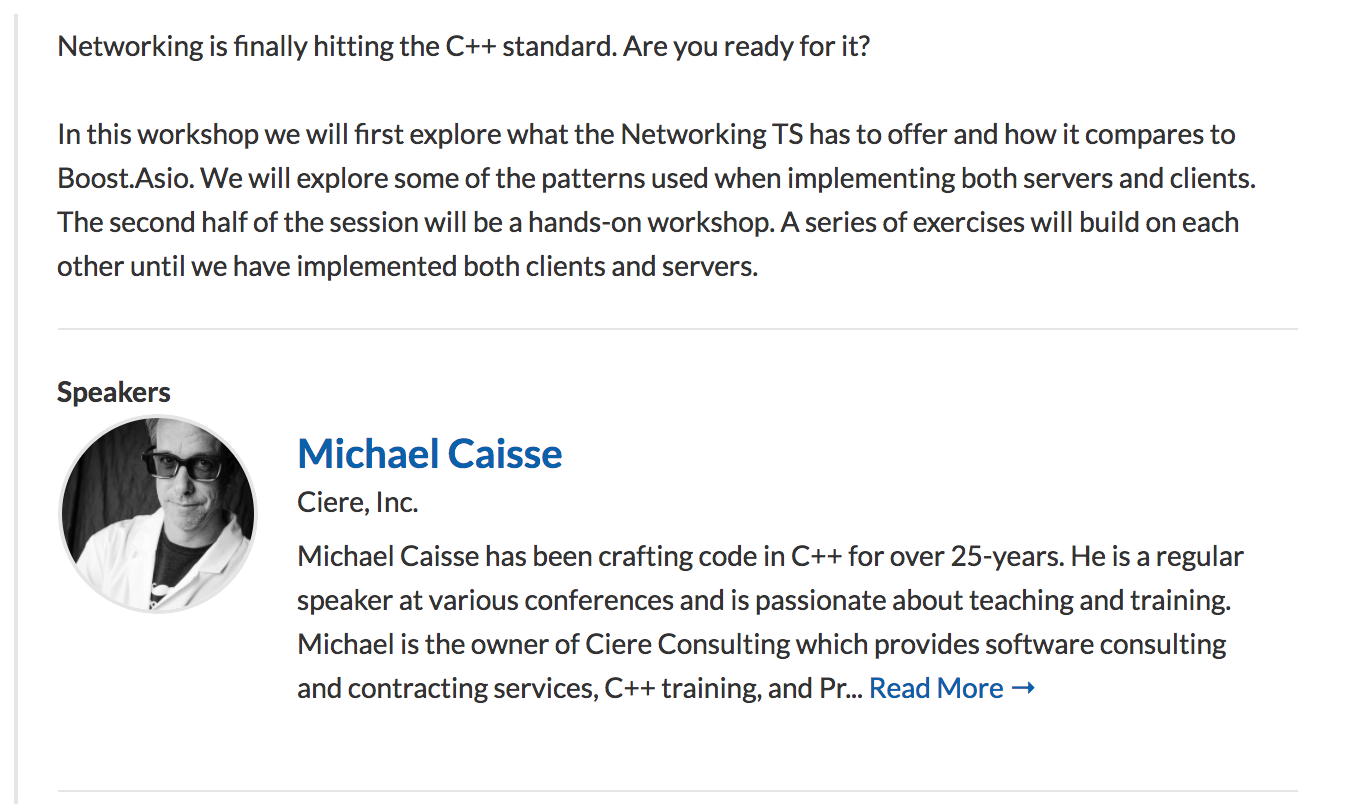 ] ] [sched](https://cppnow2017.sched.com/event/A8Ie/networking-ts-workshop-part-1-of-2) ??? > * --- # http get request ``` auto host = "www.boost.org"s; auto service = "http"s; auto request = "GET / HTTP/1.0\r\n" "Host: www.boost.org\r\n" "Accept: */*\r\n" "Connection: close\r\n\r\n"s; auto boostorg = `rx_connect`(io_context, host, service) | rxo::concat_map([=](connected c){ return rxs::just(request) | `rx_send`(c); }) | rxo::concat_map([=](connected c){ return `rx_read`(c); }) | // share chunks rxo::publish() | rxo::ref_count(); ``` ??? > * --- # derive results from the shared chunks .split-50[ .column[ .accent[ ## break out http headers] ``` auto headers = boostorg | split("\r\n", Split::RemoveDelimiter) | rxo::take_while([] (const string& s){ return !s.empty(); }); ``` ] .column[ .accent[ ## accumulate count of bytes read] ``` auto size = boostorg | rxo::scan(0, [] (int total, const string& chunk){ return total + int(chunk.size()); }); ``` ] ] ??? > * --- # combine the data sources ``` auto responseupdates = boostorg | rxo::map([](const string&){return "response"s;}); headers. concat(rxs::just("END HEADERS"s), responseupdates). with_latest_from(size | rxo::start_with(0)). ``` -- ``` subscribe(rxu::apply_to([](const string& s, int size){ cout << s << " : " << size << endl; }), [](exception_ptr ex){ cerr << rxu::what(ex) << endl; }, [](){ cout << endl; }); ``` ??? > * --- # try it out ```sh $ c++ -std=c++14 -I ../netts/include/ -I rxcpp/Rx/v2/src/ rx.cpp $ ./a.out HTTP/1.1 200 OK : 0 Date: Thu, 18 May 2017 14:03:57 GMT : 0 Server: Apache/2.2.15 (CentOS) : 0 Accept-Ranges: bytes : 0 Connection: close : 0 Content-Type: text/html : 0 END HEADERS : 0 response : 8192 response : 12288 response : 16384 response : 20480 response : 24576 response : 28672 response : 32768 response : 36864 close ``` ??? > * --- # echo ``` auto echo = rx_connect(io_context, host, service) | rxo::concat_map([=](connected c){ return rx_read(c) | rx_send(c); }) | rxo::subscribe
(); ``` ??? > * --- # code The code was a 1day minimal solution. more work is needed to build a fully general adaption. [https://github.com/kirkshoop/networkingts](https://github.com/kirkshoop/networkingts) --- # see more of these algorithms .accent[ ## No raw std::thread! - Live Tweet Analysis in C++] > * ### friday > * ### 2:30pm ??? > *